Why?
git repository is distributed in nature, also there are tons of repositories not using terraform. You have just created a killer terraform solution and cannot wait to share with world, instead of trying to send people the git repo link, how about publish it to terraform registry, and now everyone can search and simply use it as a module? After all, let’s keep it DRY (Don’t repeat yourself) as much as possible.
Integration with GitHub
Before you can publish your module to terraform, you must have a GitHub account.
Sign-in to your GitHub accout. Then navigate to https://registry.terraform.io
On top right side, click on Publish -> Module
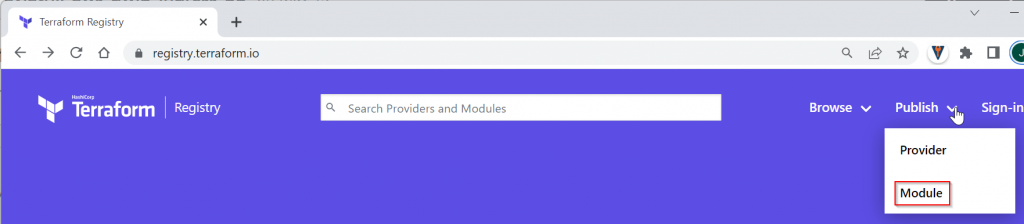
Click on Sign in with GitHub
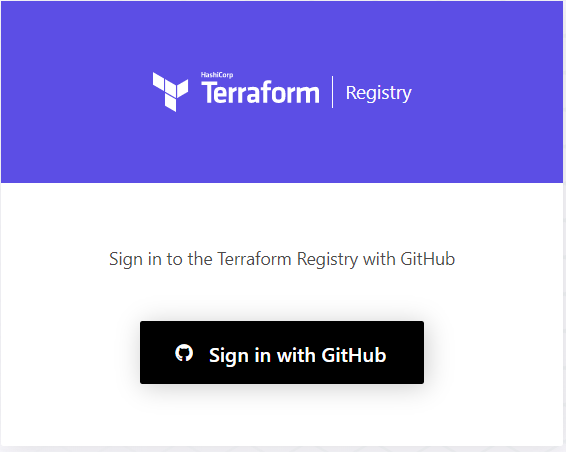
Click on the green Authorize hashicorp button
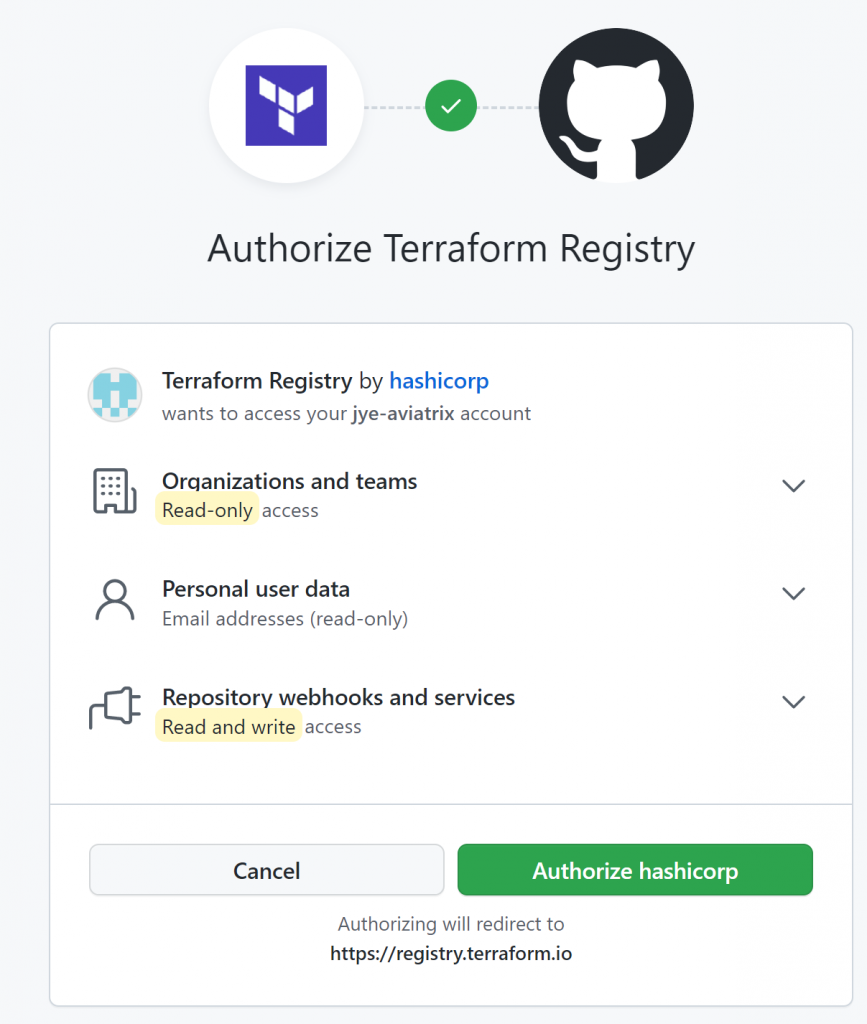
The screen returns to registry.terraform.io, but note on top right side, you have been signed in as your GitHub account.
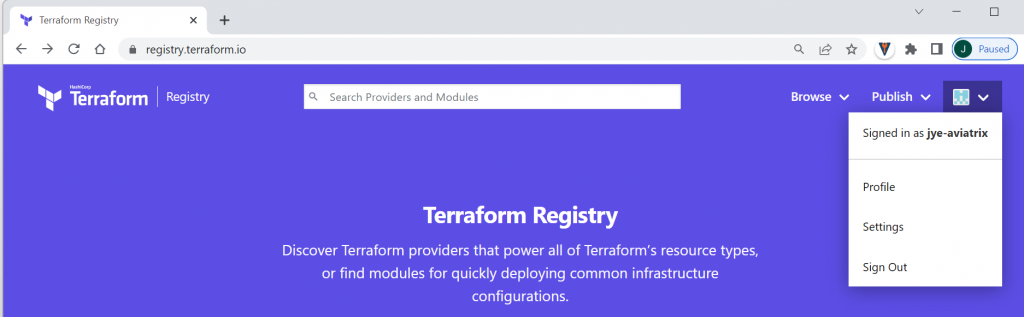
Create a test repository in GitHub
Switch to github.com Let’s create a test respository:
- Repository name format: terraform-<PROVIDER>-<NAME>. In the example, Provider = jye, Name = test
- Give it a description
- The repository must be Public
- Choose to Add a README file, terraform registry will scan and publish the content of README file automatically
- Choose .gitignore template, since we are publishing terraform module, select Terraform
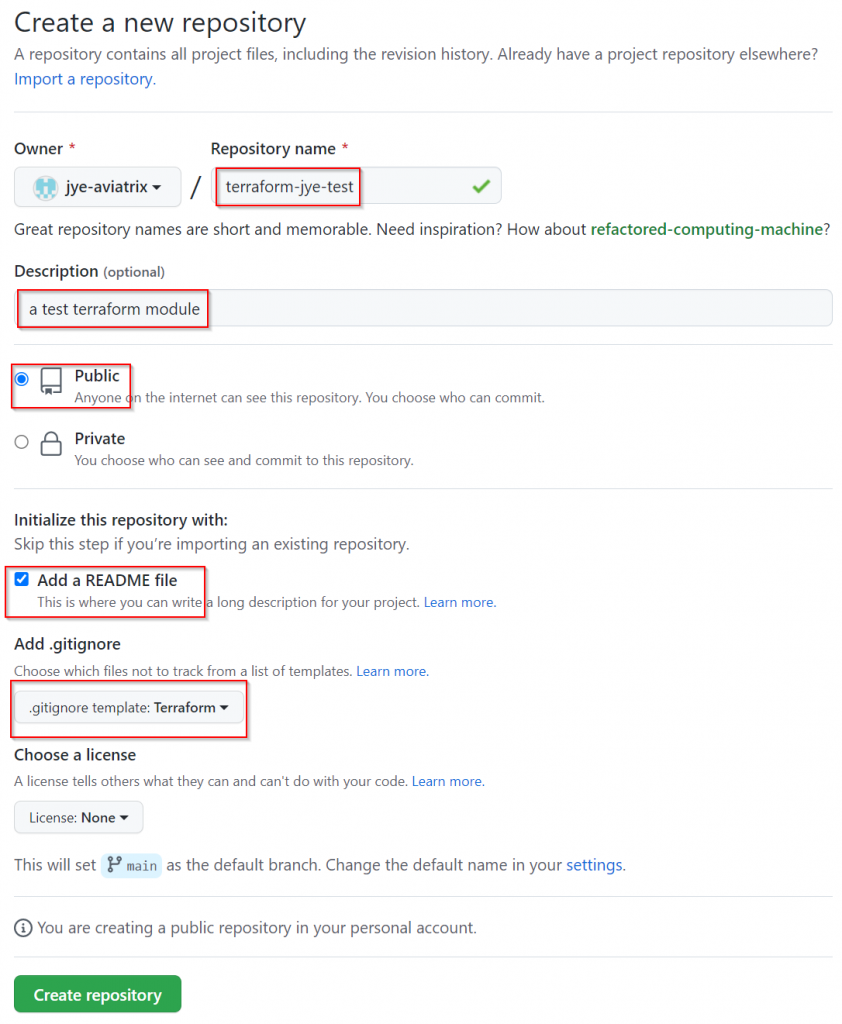
This creates an initial repository contains two files:
- .gitignore
- README.md
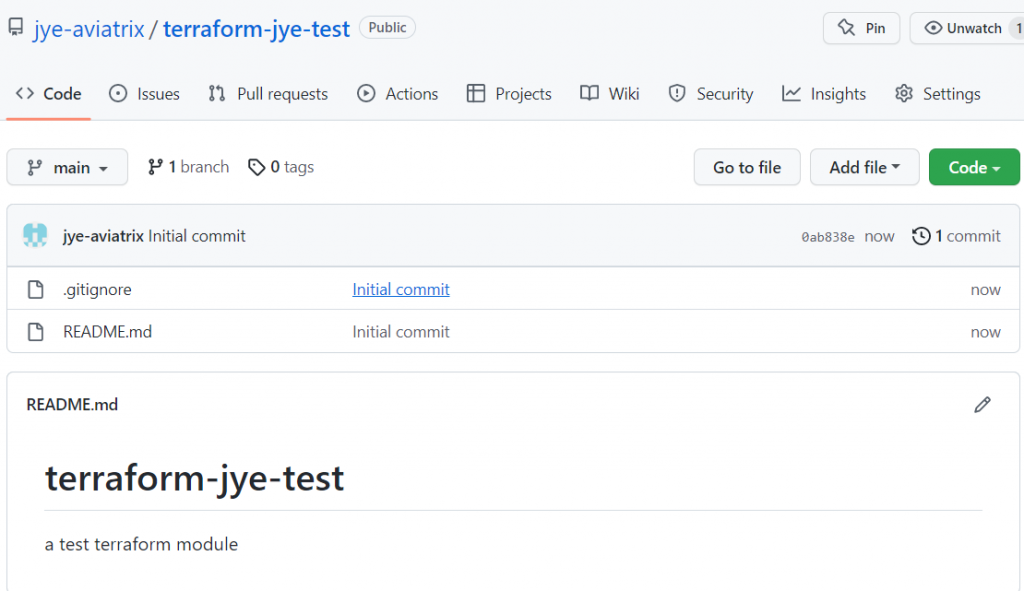
Note: You may want to spend a bit more time to decide type of license you want to apply to your code.
Publish GitHub repository to registry.terraform.io
Switch back to registry.terraform.io, on top right side, click on Publish -> Module. Only public github repo with naming format of terraform-<PROVIDER>-<NAME> will be listed here, select the repo just created. Check on I agree to the Term of Use, then click on Publish module
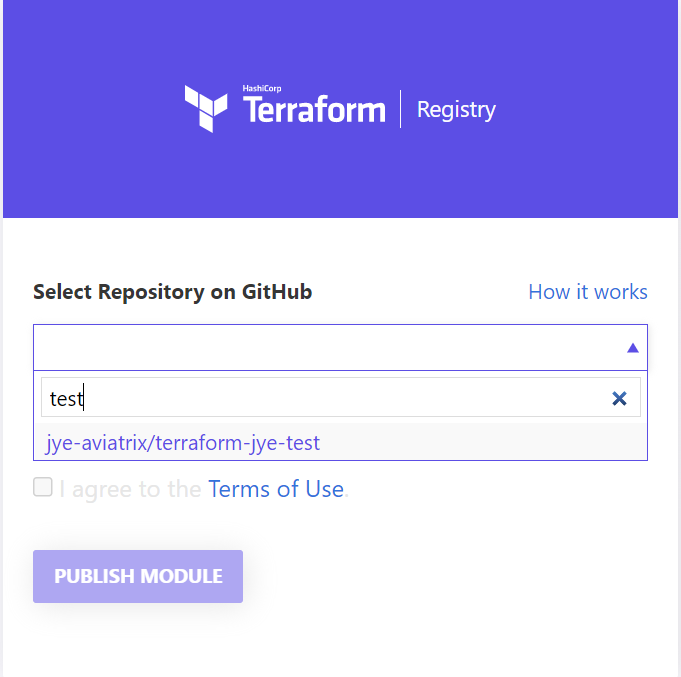
You should get an error, basically terraform registry is expecting you to provide a version/release tag that’s in the format of v1.2.3
Version number format MAJOR.MINOR.PATCH, increment the:
- MAJOR version when you make incompatible API changes
- MINOR version when you add functionality in a backwards compatible manner
- PATCH version when you make backwards compatible bug fixes
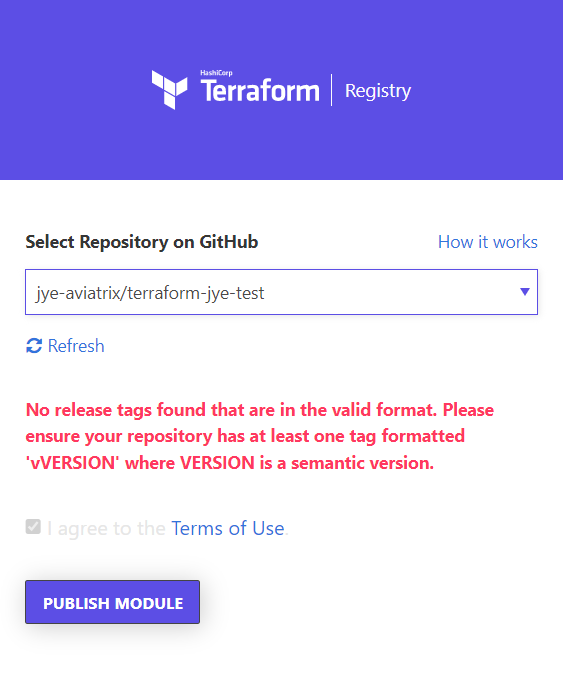
Let’s head back to GitHub.com and give our test repo a version number
Click on the 0 tags
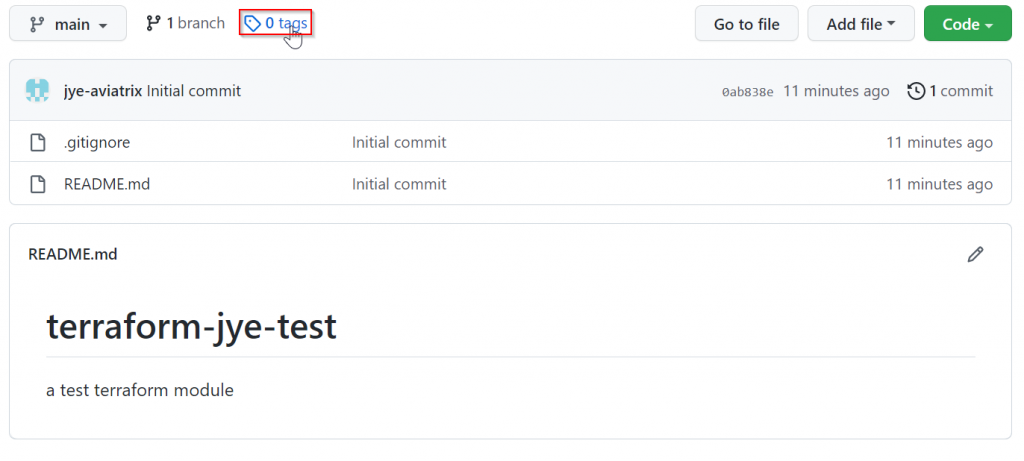
A tag requires a release, Create a new release
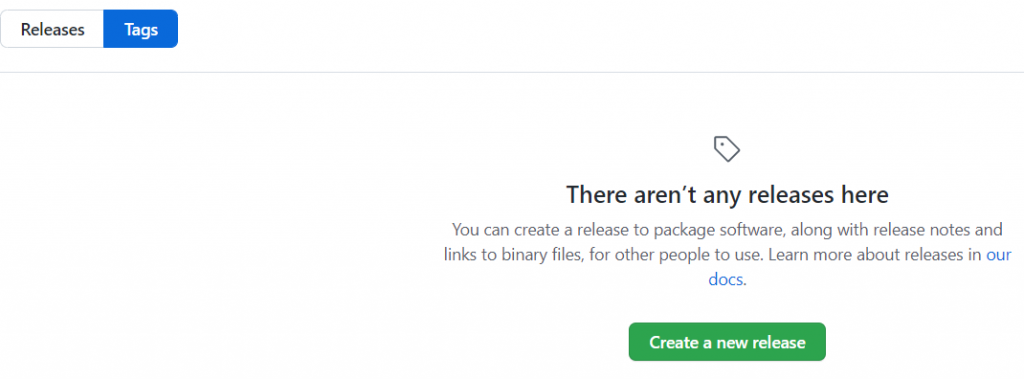
Click on Choose a tag, enter v1.0.0 as the version number, then click on Create new tag: v1.0.0 on publish
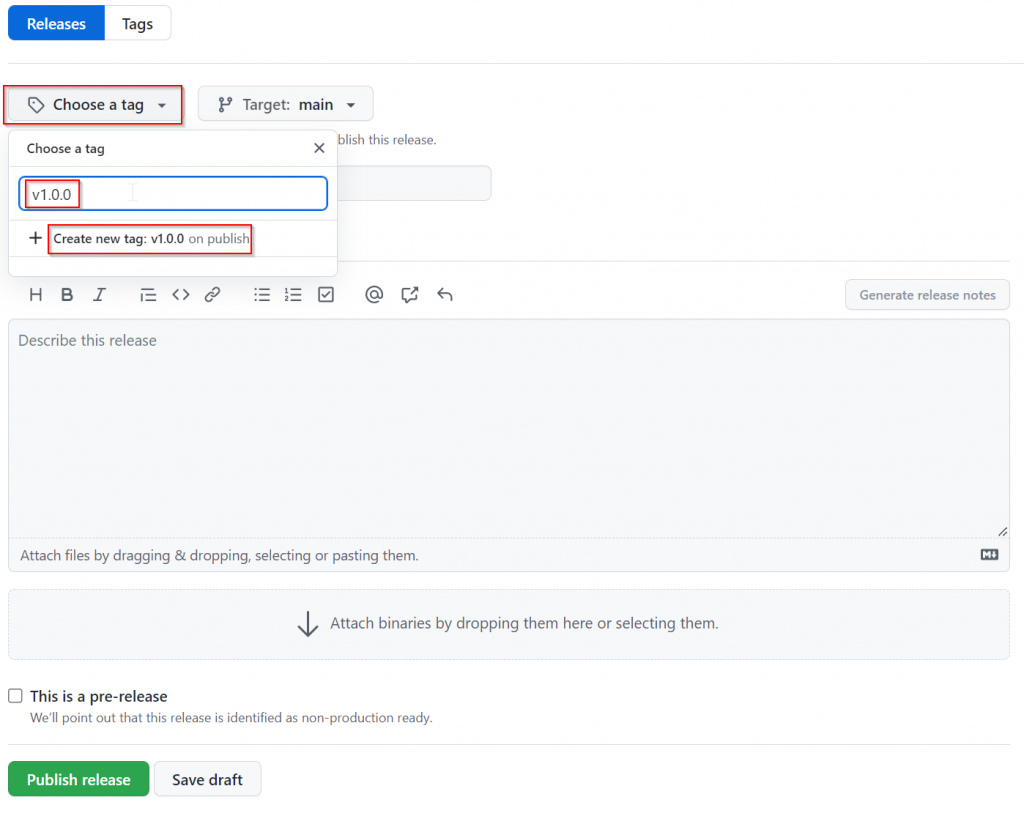
Note v1.0.0 now shows up as the tag, enter description and detail, then click on Publish release
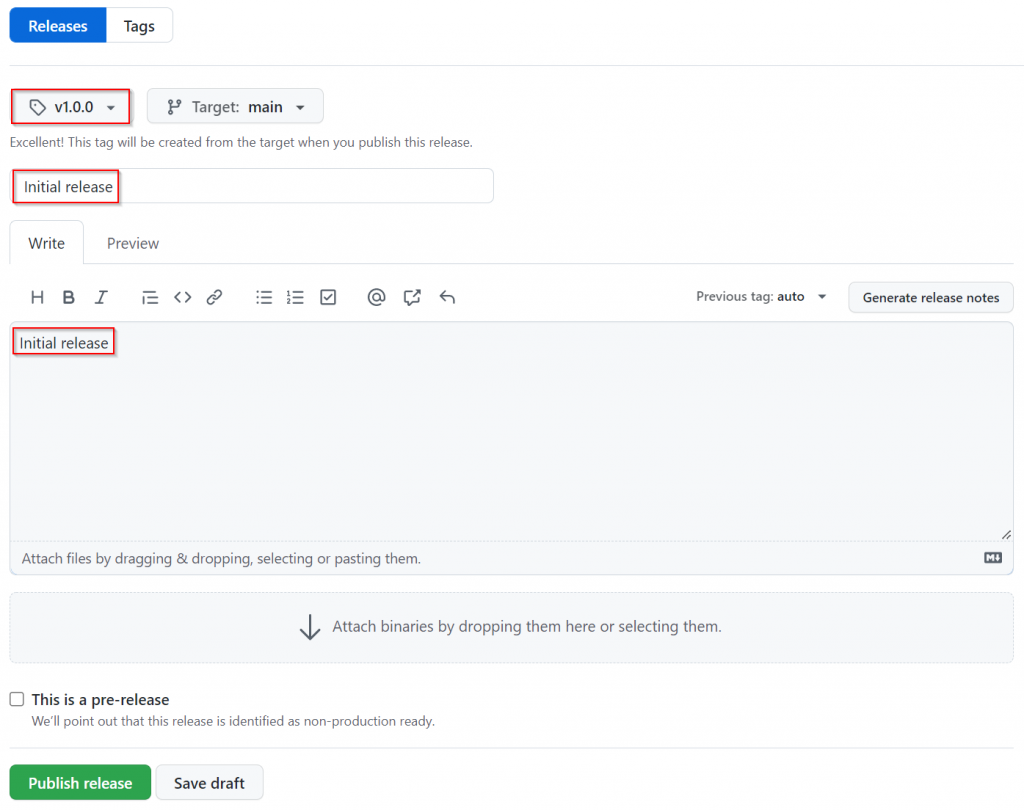
You have now created a release v1.0.0
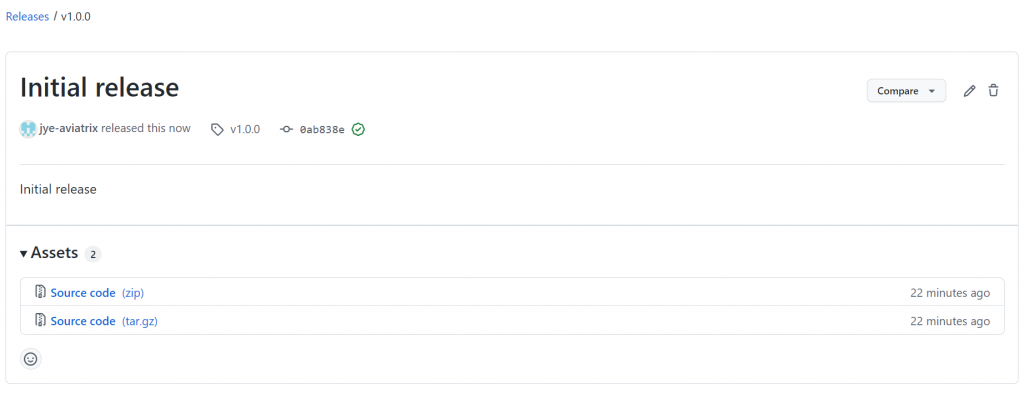
Head back to registry.terraform.io, you should still be in the screen of publishing, click Publish module again
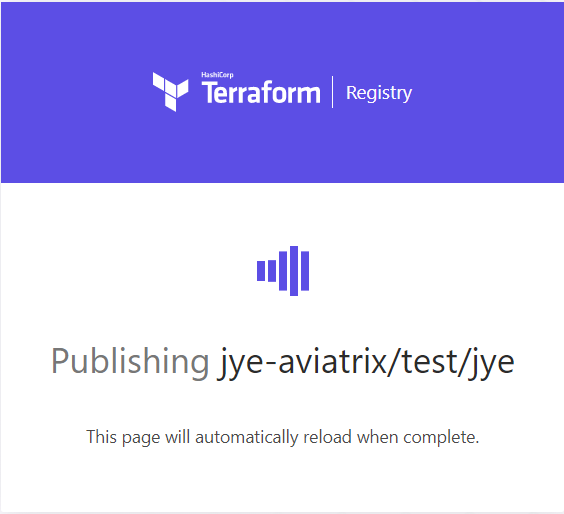
You have published your github repo to terraform registry!
Note the name of the test repository was: terraform-jye-test, you can see JYE is marked as provider, and test has been marked as name of the registry
Also note the URL is:
https://registry.terraform.io/modules/jye-aviatrix/test/jye/1.0.0
Which in the format of:
https://registry.terraform.io/modules/<github-handle>/<registry-name>/<provider-name>/<version>
Note the version is marked as Version 1.0.0, which is the current latest version
Note the content of README.md is displayed in the bottom.
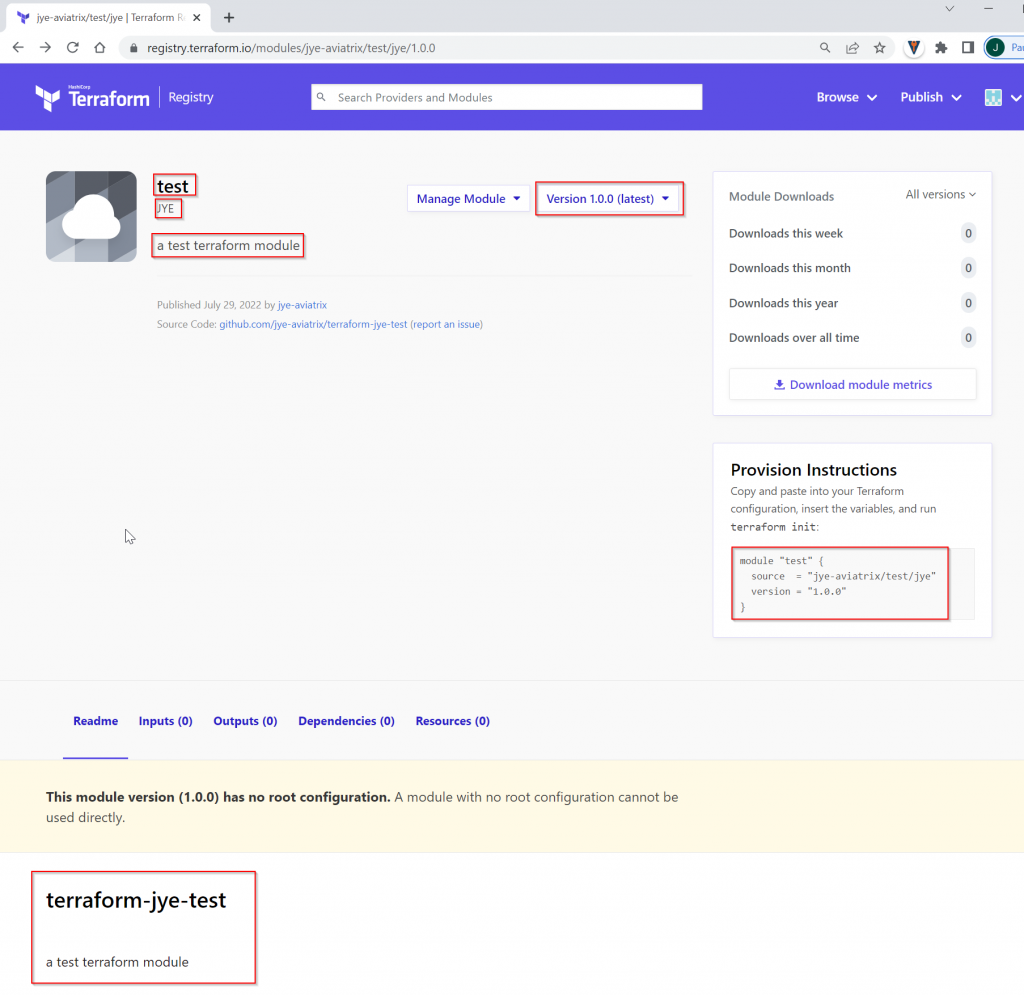
Also note that we have no terraform file created in the root directory, hence it has a yellow warning above README.
Let’s add some real code
The minimum recommended module would need following files:
README.md
main.tf
variables.tf
outputs.tf
If you want submodules or examples, consider spending a bit more time read this article about how to structure your code.
For this example, lets create the three .tf files.
variables.tf
variable "x" {
type = number
}
variable "y" {
type = number
}
main.tf
locals {
sum = var.x + var.y
}
outputs.tf
output "sum" {
value = local.sum
}
Pretty straightforward, getting the sum of input variables x and y, then write the local variable sum to output.
In your repo on github.com, click on Add file -> Create new file
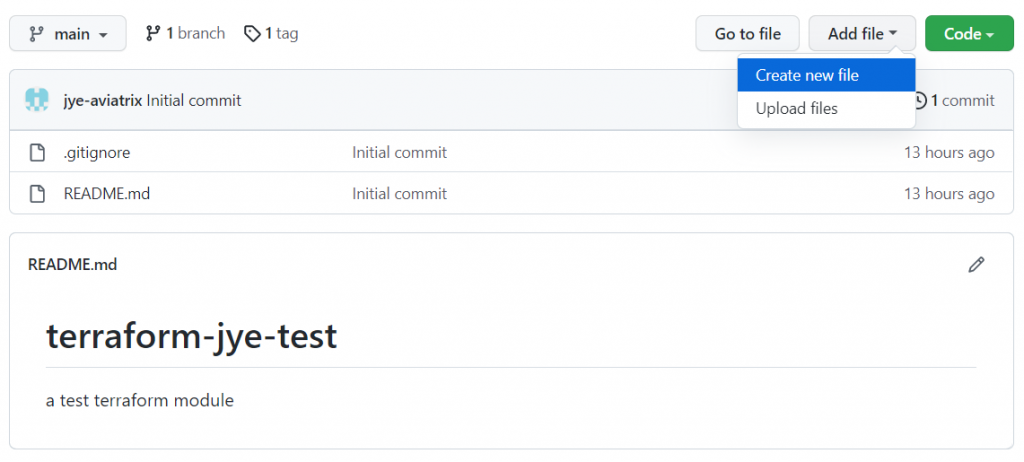
Provide file name and content of the file, as following example. Scroll down and click on Commit new file (by default directly to main branch)
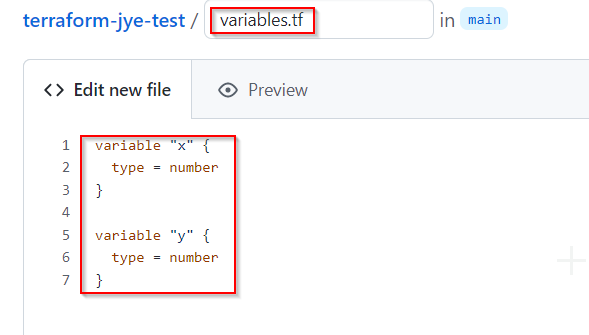
Repeat for all three files:
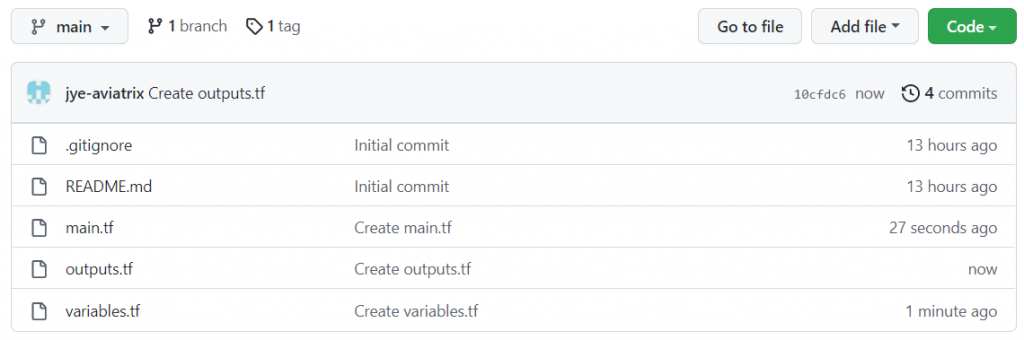
Let’s create a new release/tag v1.0.1
Click on 1 tag
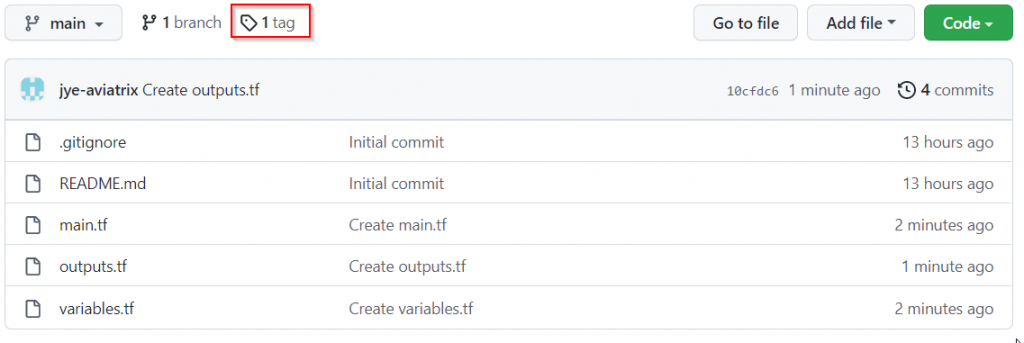
Switch to Releases tab, then click on Draft a new release
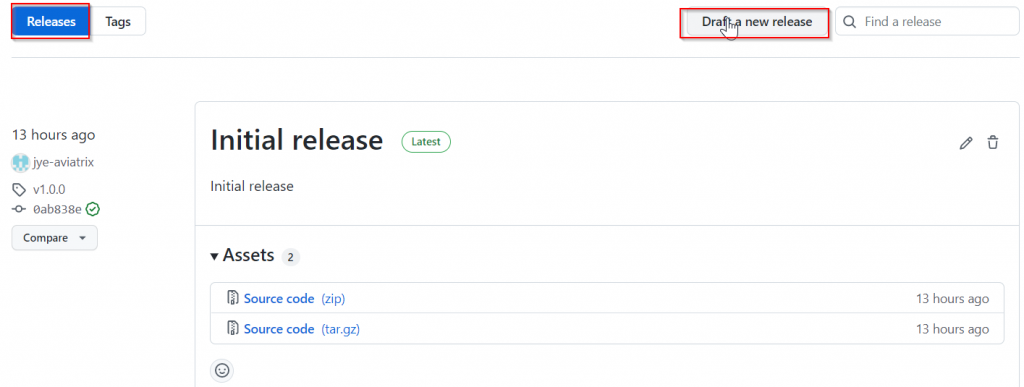
This part now is similar to what accomplished earlier, click on Choose a tag, then enter v1.0.1, then click Create new tag: v1.0.1 on publish
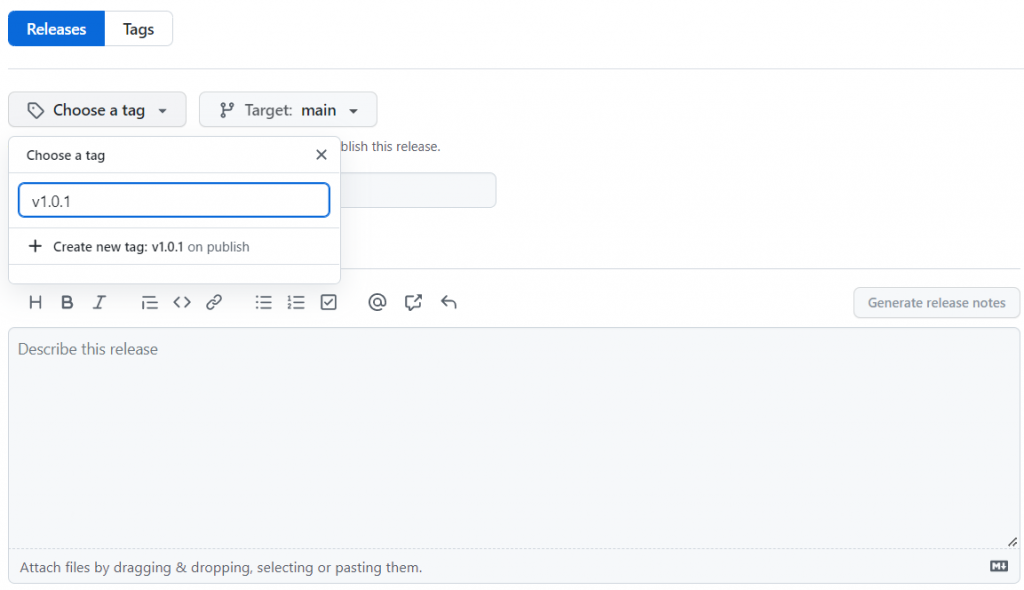
Add description and details, then click on Publish release
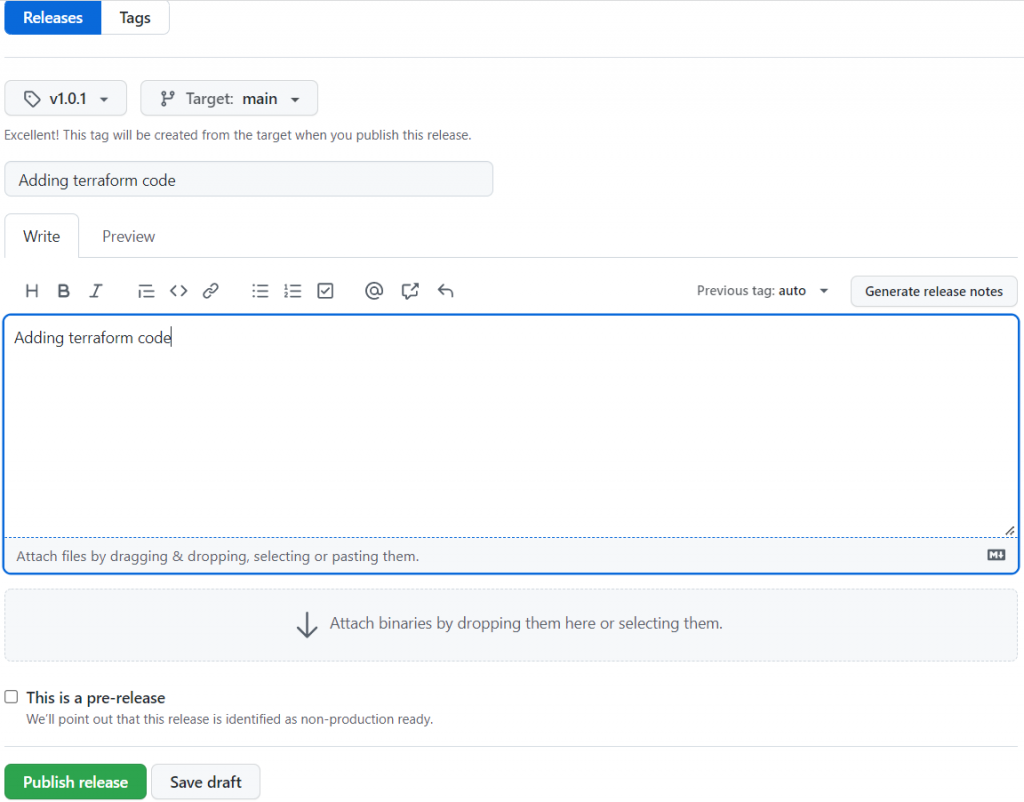
Now switch back to registry.terraform.io, you can quickly search your module by providing your github handle and module name

You will see the latest version gets updated to 1.0.1 automatically, and instruction automatically tells you to insert 2 required variables. In the bottom it lists the 2 inputs and 1 output. Nice!
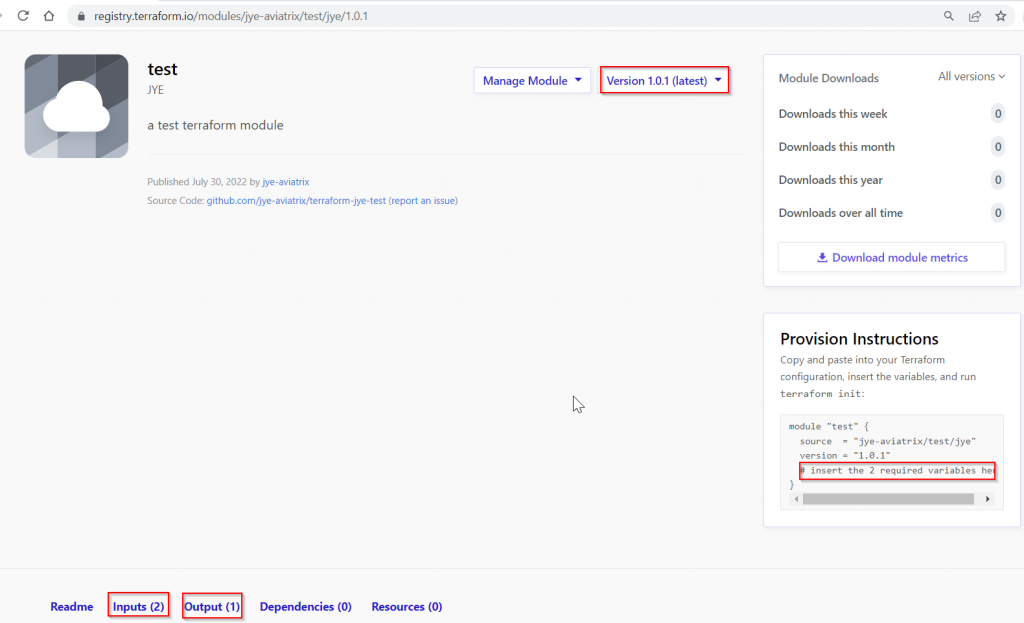
Let’s give your new module a spin!
I’m going to use Visual Studio Code for this part for easier demonstration
First create a folder called test, and populate it with main.tf
Copy the Provision instructions code from registry.terraform.io to VS Code
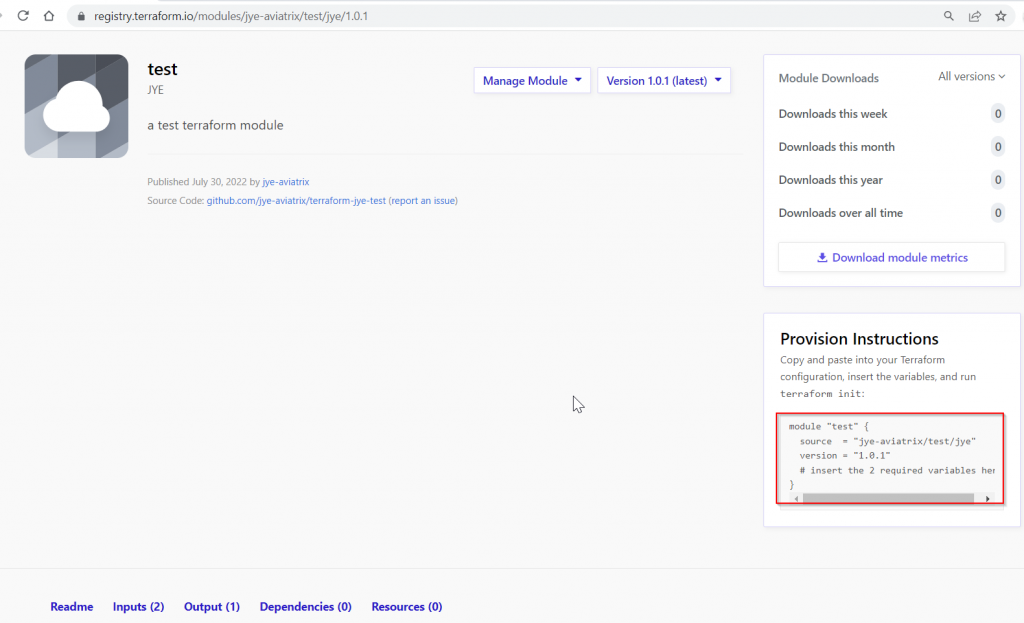
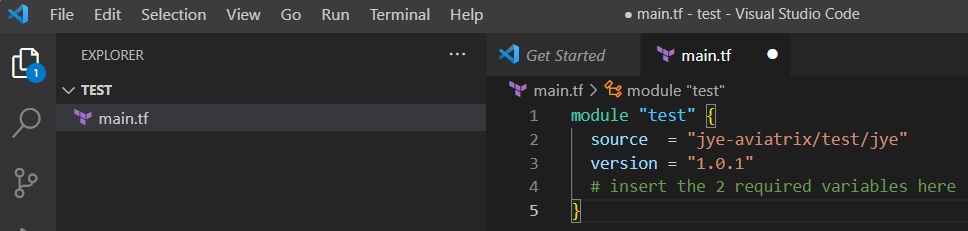
Look at inputs section, and enter the two inputs in VS Code
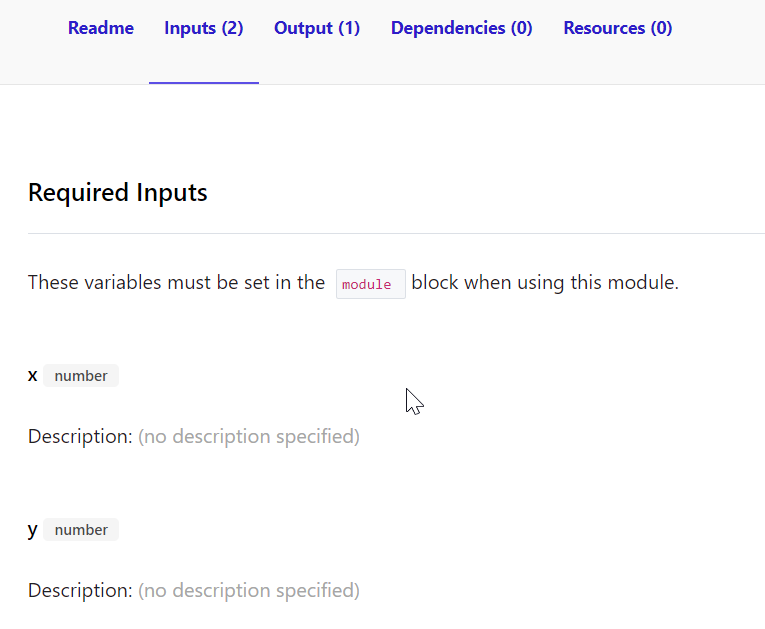

Your code would look like this:
module "test" {
source = "jye-aviatrix/test/jye"
version = "1.0.1"
# insert the 2 required variables here
x = 20
y = 6
}
Add outputs.tf, it references the output sum from the module
output "sum" {
value = module.test.sum
}
Run: terraform init
Since we didn’t specify any version constrain, it automatically downloaded latest version
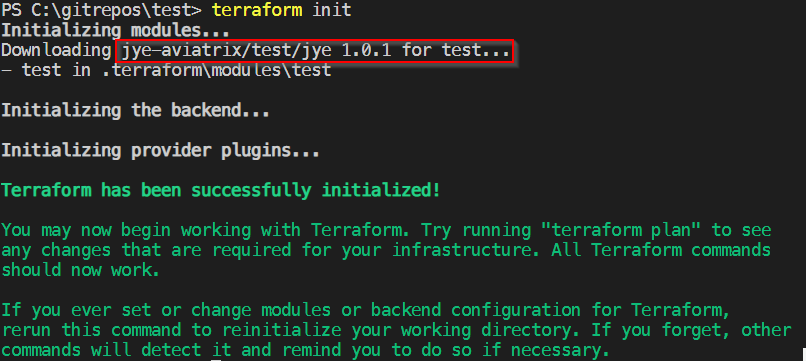
Then run: terraform apply –auto-approve
Looking great!
